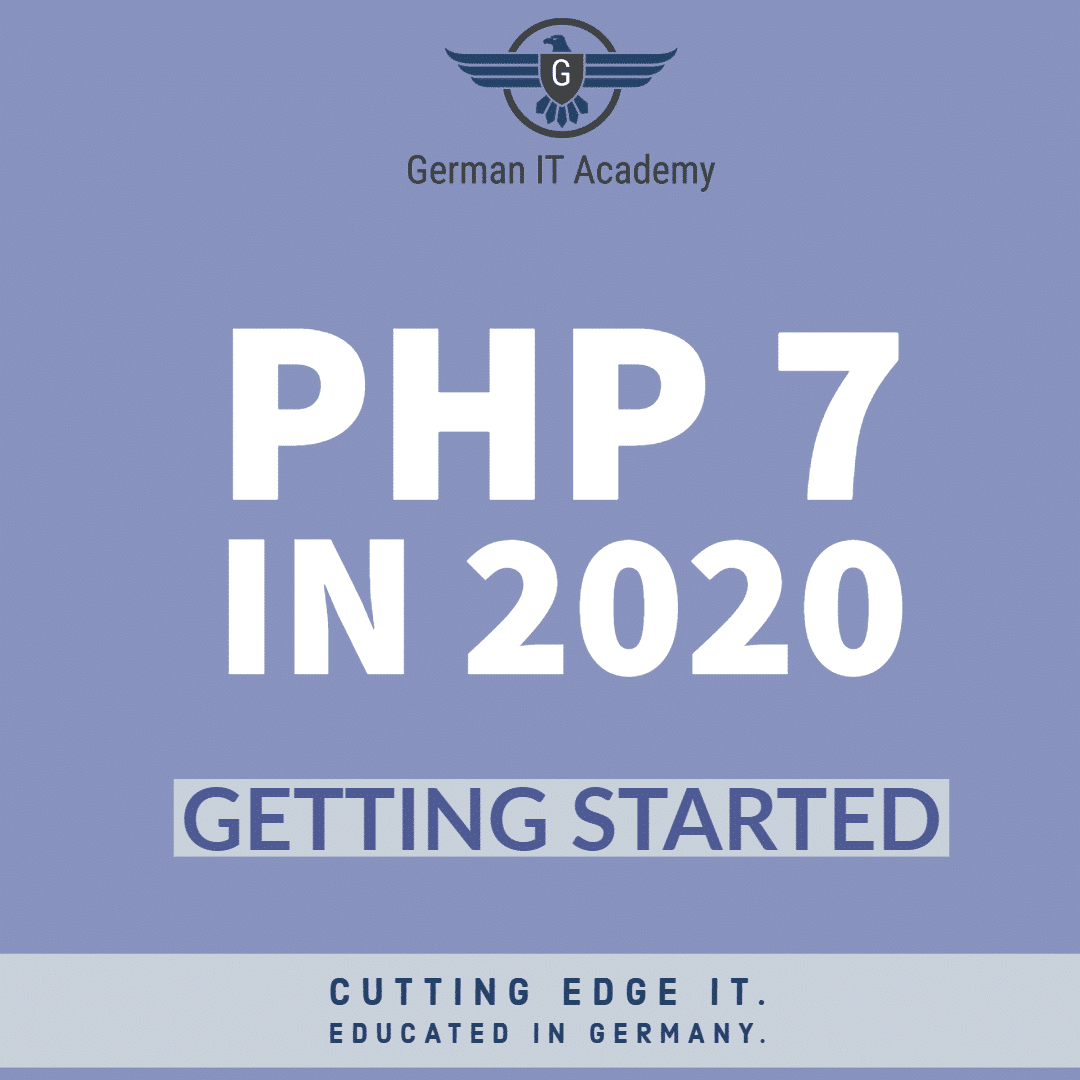
Getting started with PHP 7 in 2020
What is PHP?
PHP stands for Hypertext Preprocessor (earlier called „Personal home page“). It is an open source scripting language created for the web development. PHP is a server-side language which means all the code is rendered on server and only static HTML, CSS and JavaScript is sent to the client’s browser. PHP is a back-end language which means no one on client side can check the code written in PHP, only the person with authority to access the server can check the source code. Now let’s explore reasons to learn PHP 7 in 2020 and what lies ahead for this simple and beautiful language.
Why should I learn PHP 7 in 2020?
PHP is compatible with all leading operating systems such as Windows, Linux, MAC OS and many others. Likewise, it is compatible with all popular web servers such as Apache, NGINXm IIS etc. PHP7 offers great compatibility and performance. Not only is it open source but also it contains many features and it is absolutely easy to install and set-up. We will cover installation part later in this article.
What are features of PHP?
PHP comes with many benefits that let you create wonderful web applications.
1. Open Source
PHP is an open source programming language which means it is free to use. There is a great community to help you if you get stuck anywhere and not able to figure out the problem.
2. Cross-Platform
PHP supports all major operating systems and servers.
3. Easy to use
PHP is simple and clean scripting language. Its syntax is convenient to use. Anyone can quickly learn PHP.
4. Performance
It is one of the fastest programming language. It takes so little time to establish a connection with database or fetch data from web services.
How to install PHP7?
You can follow this guide to install PHP7 in your preferred operating system.
Windows :
Step 1 – Download PHP7
Go to the official PHP website and download the latest version. Save it on your windows PC.
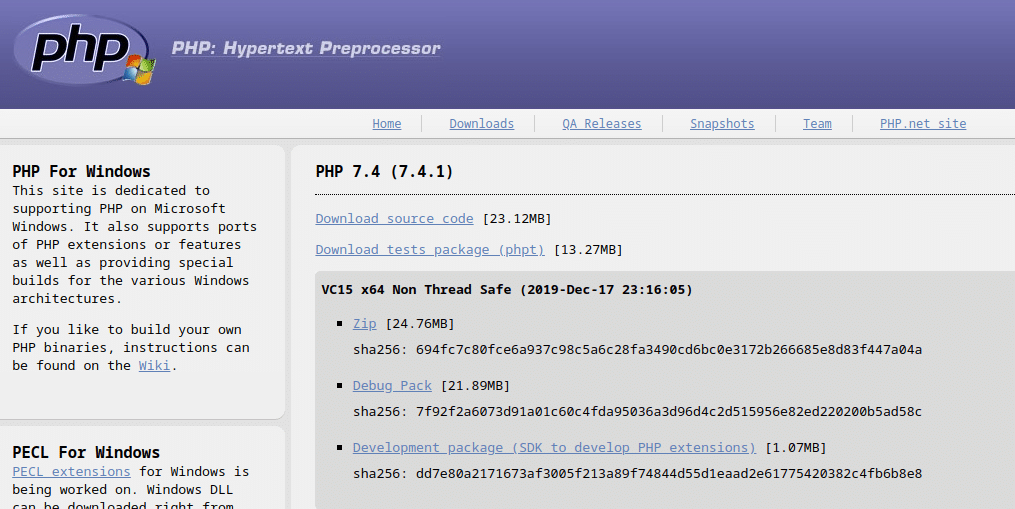
Step 2 – Extract the zip
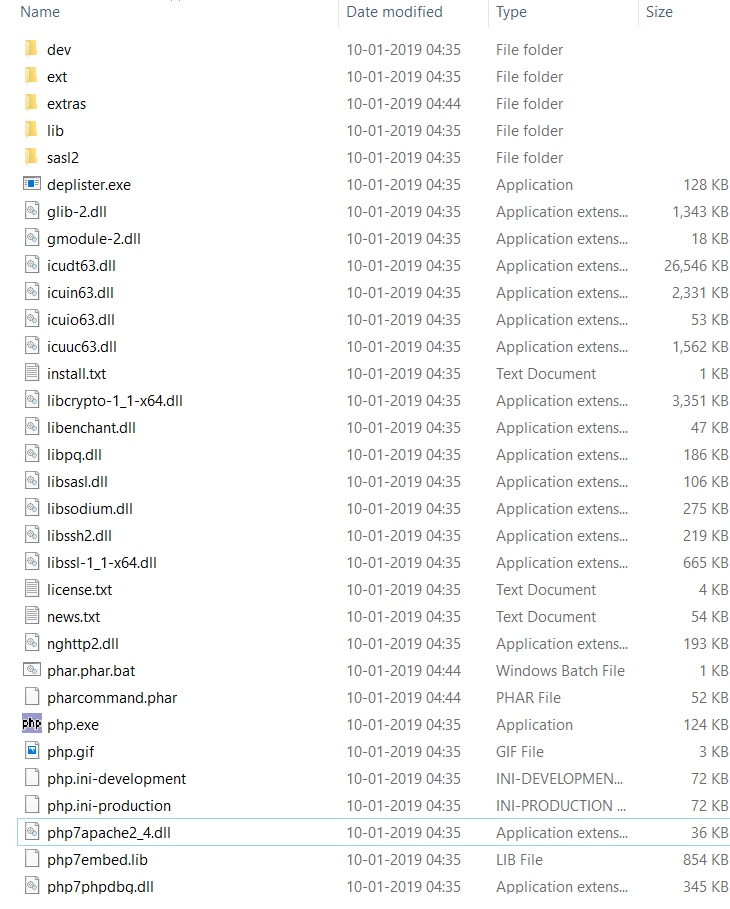
Step 3 – Setting up Environment variable
We need to configure the environment variable to access PHP from the command line. You can follow these steps to do so.
Right click -> My Computer (This PC) -> Properties -> Advanced system settings
This will open a the following window.
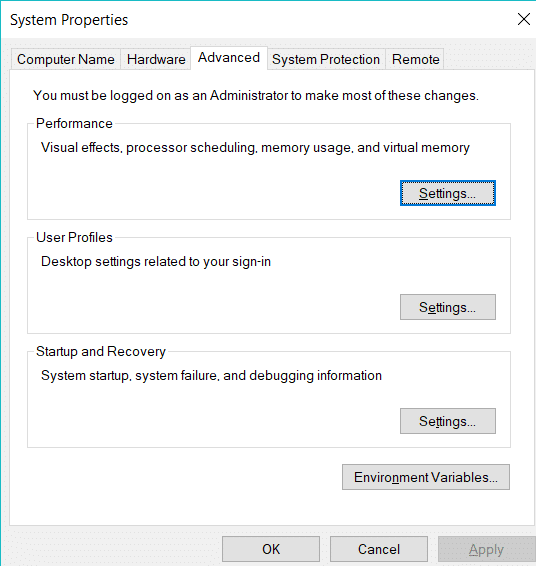
Now click on Environment variables, select path under the System variables section and edit. Add the path of installed PHP to system path.
Click on the new button and add the path to the PHP bin. Save all changes and close all the windows. Now to verify the settings, open console (or CMD) and execute following.
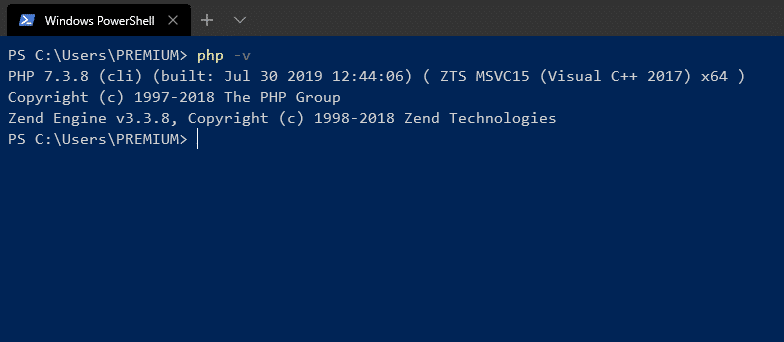
If you get the following output, it means PHP is successfully installed on Windows.
Linux:
Here, we will see the steps to install PHP in Ubuntu Linux.
Step 1 – Update Ubuntu
Before installing anything new, you should always update your system. Open a terminal and run the following commands:
apt-get upgrade && apt-get update
Step 2 – Install PHP7
You can install PHP7 from Ubuntu’s official repository by following command:
apt-get install php
This command will install PHP7 and it’s required dependencies. To check whether PHP is installed or not execute following command:
php -v
It will return the PHP version related information.
PHP7 Syntax Overview
Like any other programming language PHP has a set of syntax rules which we need to follow while programming. PHP files commonly have a .php file extension as they’re always executed on server.
Tags in PHP or Escaping to PHP
The PHP parsing engine needs a way to differentiate PHP code from other elements. PHP tags are used for this.
We can ‘Escape to PHP’ by using the following 4 methods:
- Canonical PHP tags
- SGML (or) Short HTML Tags
- HTML Script Tags
- ASP Style Tags
From all of the above, Canonical PHP tags are most commonly used and are compatible with majority of servers.
Example:
<?php
# Here the echo command prints the message
echo "Hello world";
?>
Comments in PHP
Comments are statements in a program by developers which describe the intent and purpose of the code. There are 2 styles for comments in PHP. Comments in PHP code are not read or executed by the PHP engine. Therefore, they do not affect the output.
Single Line Comment
<?php
// This is a single line comment
echo "Hello World";
# This is also a single line comment
?>
Multi line Comment
<?php
/*
Example for multiline comment
This is a multiline comment
*/
echo "Hello world";
?>
PHP Syntax is Case-Sensitive
In PHP besides variables, all other keywords are not case-sensitives. We have to be very careful while defining and using variables. Look at this example.
<?php
// Here all echo statements will execute
// in the same manner because keywords are case-insensitive
$variable = "Test";
ECHO $variable;
// You will get an "Undefined Variable" error
// Because variable names are case-sensitives
echo $VARIABLE;
?>
If you want to build a fast and beautiful Frontend for you PHP-Backend Application, consider the popular Framework Vue.JS 2 Course.
-
Vue.js 2Produkt im Angebot29,00 €
PHP Data types & Variables
Data Types
A Data type is the classification of data into a category according to its attributes. There are 4 major data types in PHP.
- Integer – eg. 1, 1000, -12
- Float – eg. 3.14
- String – eg. „Test String“
- Boolean – eg. True or False
Variables
A variable is a name given to a memory location that stores data at runtime. There are 2 types of variables, Global variables and Local variables. There are some pre-defined rules to create variables.
- All variable names must start with the dollar sign. – eg. $testVariable
- Variable names are case sensitive.
- All variable names must start with a character.
- Variable names must not contain any whitespaces between them.
PHP Control Structure – If else
If else is the simplest control structure. And it is relatively easy to understand even in PHP 7 in 2020. It evaluates the conditions using Boolean logic. Take a look at the following code snippet:
<?php
if (condition is true) {
// Code block one
}else {
// Code block two
}
?>
Here,
- „(condition is true)“ is the control structure, if it evaluates to true, „Code Block one“ will be executed
- If condition evaluates to false, „Code block two“ will be executed.
- In any situation only one of the following blocks will be executed.
<?php
// If else example
$x = 10;
$y = 20;
if($x > $y) {
echo "$x is greater than $y";
} else {
echo "$y is greater than $x";
}
// Output: 20 is greater than 10
?>
PHP Loops
While Loop
The while loop is use to execute a block of code repeatedly until the codition gets statisfied.
<?php
while (condition) {
// Code to execute
}
?>
Here,
- Code inside of while loop will be executes until the given „condition“ is true.
<?php
$i = 0;
while ($i < 5) {
echo $i;
$i++;
}
/*
Output:
0
1
2
3
4
*/
?>
For Loop
For loop executes the block of code a specified number of times.
<?php
To (initialization; condition; increment/decrement) {
// Code to be executed
}
?>
Here,
- „initialize“ is used to set the counter’s initial value.
- „condition“ is the condition that is evaluated for each loop iteration.
- „increment/decrement“ is used to increment or decrement the counter.
<?php
for ($i = 0; $i < 5; $i++) {
echo $i;
}
/*
Output:
0
1
2
3
4
*/
?>
ForEach Loop
The php foreach loop is used to iterate through array values.
<?php
foreach($array_variable as $array_value){
//Code block to be executed
}
?>
Here,
- $array_variable is the array variable to be looped through.
- $array_value is the temporary variable that holds the current array item.
<?php
$arr = [1, 2, 3, 4, 5]
foreach ($arr as $item) {
echo $item;
}
/*
Output:
1
2
3
4
5
*/
?>
Executing PHP File
There are two ways to execute PHP files.
- Executing PHP file in browser
- Executing PHP file in terminal (command line)
Executing PHP file in browser
Step 1: Create your PHP file in www or htdocs folder of your server.
Step 2: Turn on your server.
Step 3: Go to http://localhost/<file_name>
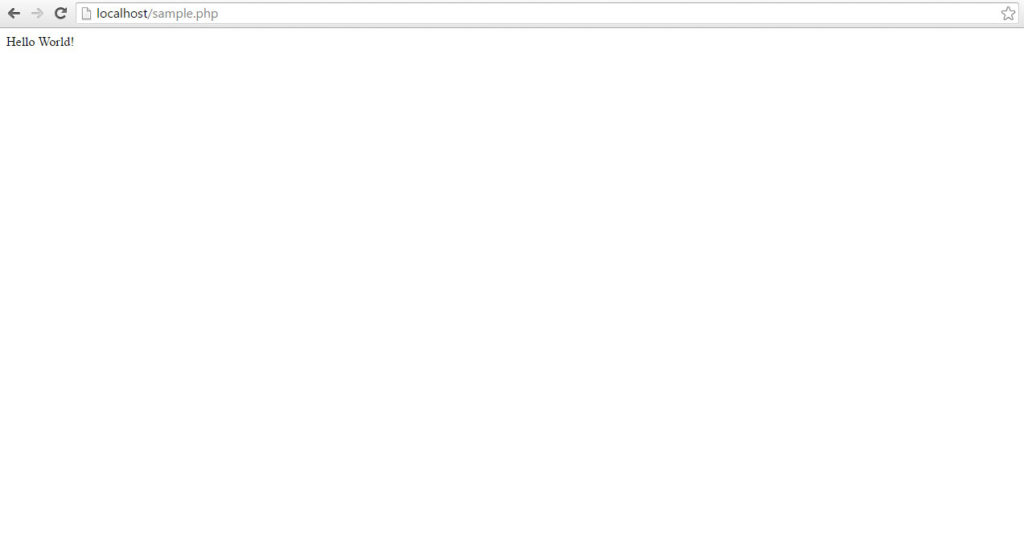
Executing PHP file from terminal (command line)
Step 1: Open your preferred terminal
Step 2: Navigate to your file location (directory)
Step 3: Execute the file by following command
php <filename.php>
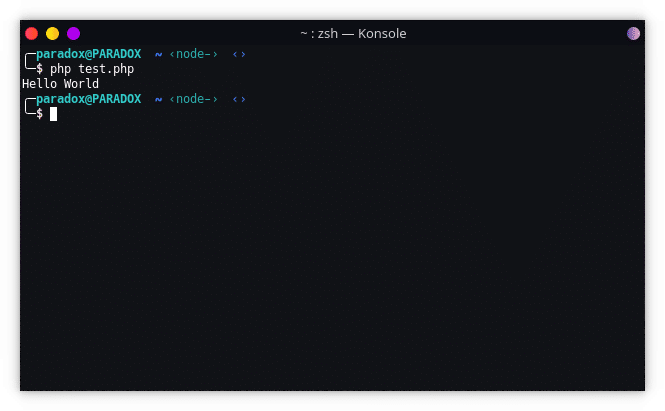
As you can see, it will one of the best skills you could acquire for yourself and your resume. We are certain that PHP 7 in 2020, will have a continuously growing community.